While and Do...While Loop
C Programming while and do...while Loop
Loops are used in programming to repeat a specific block of code. After reading this tutorial, you will learn how to create a while and do...while loop in C programming.
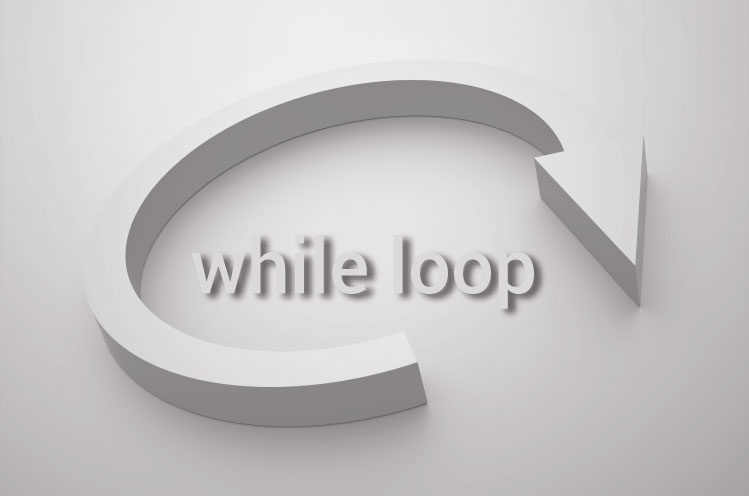
Loops are used in programming to repeat a specific block until some end condition is met. There are three loops in C programming:
- for loop
- while loop
- do...while loop
while loop
The syntax of a while loop is:
while (testExpression) { //codes }
where,
testExpression
checks the condition is true or false before each loop.How while loop works?
The while loop evaluates the test expression.
If the test expression is true (nonzero), codes inside the body of while loop are exectued. The test expression is evaluated again. The process goes on until the test expression is false.
When the test expression is false, the while loop is terminated.
Flowchart of while loop
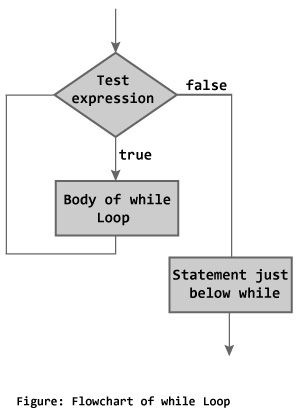
Example #1: while loop
// Program to find factorial of a number
// For a positive integer n, factorial = 1*2*3...n
#include <stdio.h>
int main()
{
int number;
long long factorial;
printf("Enter an integer: ");
scanf("%d",&number);
factorial = 1;
// loop terminates when number is less than or equal to 0
while (number > 0)
{
factorial *= number; // factorial = factorial*number;
--number;
}
printf("Factorial= %lld", factorial);
return 0;
}
Output
Enter an integer: 5 Factorial = 120
To learn more on test expression (when test expression is evaluated to nonzero (true) and 0 (false)), check out relational and logical operators.
do...while loop
The
do..while
loop is similar to the while
loop with one important difference. The body of do...while
loop is executed once, before checking the test expression. Hence, the do...while loop
is executed at least once.do...while loop Syntax
do { // codes } while (testExpression);
How do...while loop works?
The code block (loop body) inside the braces is executed once.
Then, the test expression is evaluated. If the test expression is true, the loop body is executed again. This process goes on until the test expression is evaluated to 0 (false).
When the test expression is false (nonzero), the
do...while
loop is terminated.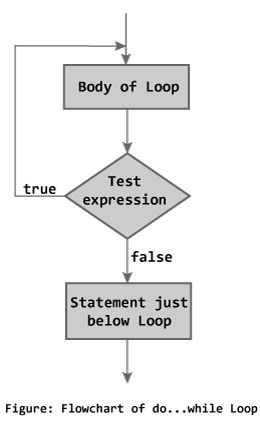
Example #2: do...while loop
// Program to add numbers until user enters zero
#include <stdio.h>
int main()
{
double number, sum = 0;
// loop body is executed at least once
do
{
printf("Enter a number: ");
scanf("%lf", &number);
sum += number;
}
while(number != 0.0);
printf("Sum = %.2lf",sum);
return 0;
}
Output
Enter a number: 1.5 Enter a number: 2.4 Enter a number: -3.4 Enter a number: 4.2 Enter a number: 0 Sum = 4.70
To learn more on test expression (when test expression is evaluated to nonzero (true) and 0 (false)), check out relational and logical operators.
Comments
Post a Comment