C break and continue Statement
C Programming break and continue Statement
In this tutorial, you will learn how to use break and continue statements to alter the program flow of loops.
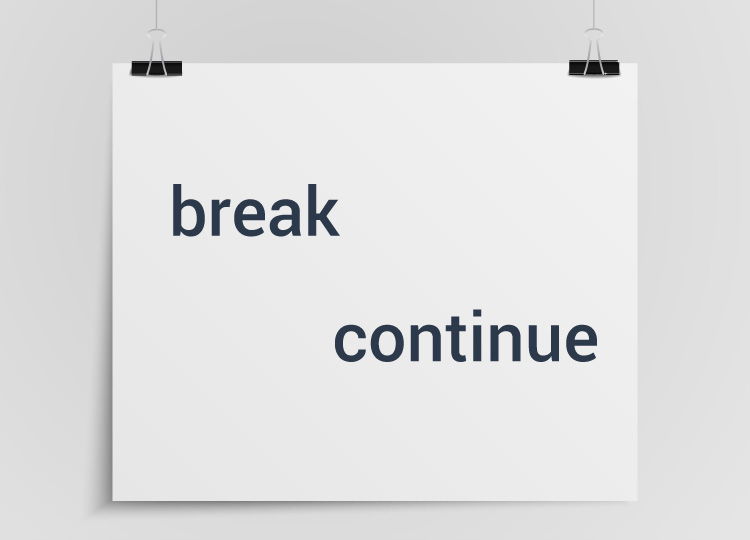
It is sometimes desirable to skip some statements inside the loop or terminate the loop immediately without checking the test expression.
In such cases,
break
and continue
statements are used.break Statement
The break statement terminates the loop (for, while and do...while loop) immediately when it is encountered. The break statement is used with decision making statement such as if...else.
Syntax of break statement
break;
The simple code above is the syntax for break statement.
Flowchart of break statement
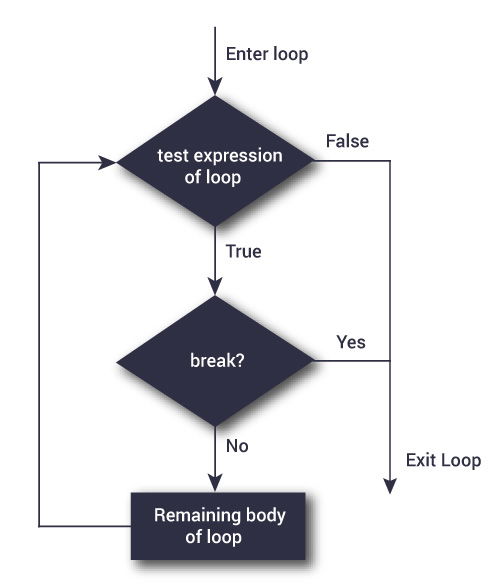
How break statement works?
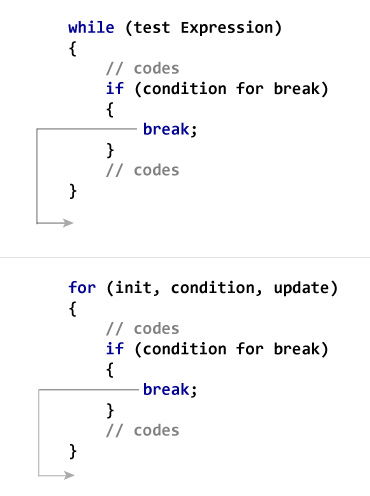
Example #1: break statement
// Program to calculate the sum of maximum of 10 numbers
// Calculates sum until user enters positive number
# include <stdio.h>
int main()
{
int i;
double number, sum = 0.0;
for(i=1; i <= 10; ++i)
{
printf("Enter a n%d: ",i);
scanf("%lf",&number);
// If user enters negative number, loop is terminated
if(number < 0.0)
{
break;
}
sum += number; // sum = sum + number;
}
printf("Sum = %.2lf",sum);
return 0;
}
Output
Enter a n1: 2.4 Enter a n2: 4.5 Enter a n3: 3.4 Enter a n4: -3 Sum = 10.30
This program calculates the sum of maximum of 10 numbers. It's because, when the user enters negative number, the break statement is executed and loop is terminated.
In C programming, break statement is also used with switch...case statement.
continue Statement
The continue statement skips some statements inside the loop. The continue statement is used with decision making statement such as if...else.
Syntax of continue Statement
continue;
Flowchart of continue Statement
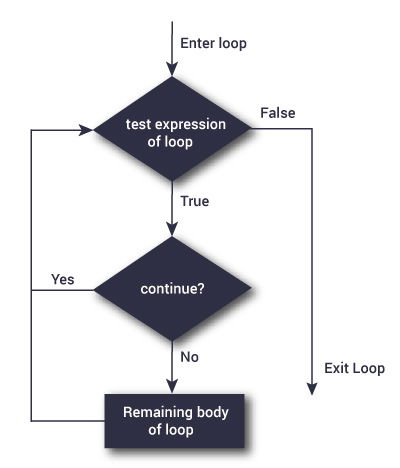
How continue statement works?
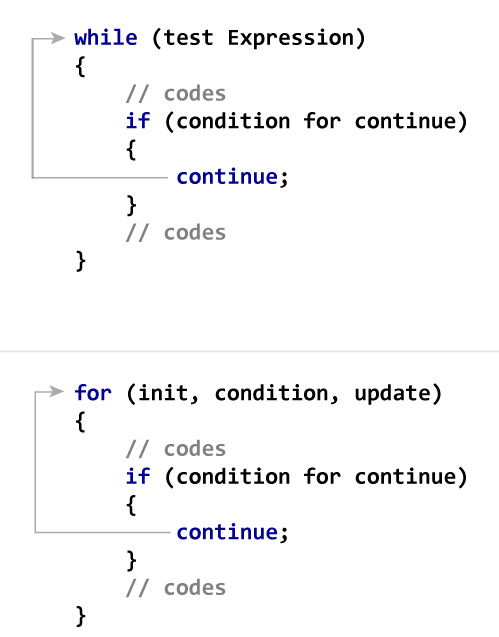
Example #2: continue statement
// Program to calculate sum of maximum of 10 numbers
// Negative numbers are skipped from calculation
# include <stdio.h>
int main()
{
int i;
double number, sum = 0.0;
for(i=1; i <= 10; ++i)
{
printf("Enter a n%d: ",i);
scanf("%lf",&number);
// If user enters negative number, loop is terminated
if(number < 0.0)
{
continue;
}
sum += number; // sum = sum + number;
}
printf("Sum = %.2lf",sum);
return 0;
}
Output
Enter a n1: 1.1 Enter a n2: 2.2 Enter a n3: 5.5 Enter a n4: 4.4 Enter a n5: -3.4 Enter a n6: -45.5 Enter a n7: 34.5 Enter a n8: -4.2 Enter a n9: -1000 Enter a n10: 12 Sum = 59.70
In the program, when the user enters positive number, the sum is calculated using
sum += number;
statement.
When the user enters negative number, the
continue
statement is executed and skips the negative number from calculation.
Comments
Post a Comment